Abstract
This research paper addresses the analysis of user relationships within social networks using Graph Neural Networks (GNN). A GNN model was applied to a social network derived from Facebook user data, analyzing the links between users to understand the social structure of the community. The results showed the effectiveness of using GNNs for social community detection, contributing to the development of social network analysis strategies.
1. Introduction
In the digital information age, understanding the relationships between users in social networks is increasingly important. This study analyzes the Facebook social network using deep learning techniques, specifically Graph Neural Networks, which have proven effective in processing structural data.
2. Previous Work
Numerous studies have addressed social network analysis, most utilizing various techniques such as traditional machine learning. However, the use of Graph Neural Networks represents a significant advancement in this field, as this technique can better exploit structural information.
3. Data and Sources
Link data was extracted from the Facebook network. Link data was loaded from the `facebook_combined.txt` file, which contains information about user links.
4. Methodology
4.1 Data Preparation
A network was created using the NetworkX library, and data was prepared to fit the requirements of PyTorch Geometric.
4.2 GNN Model
A GNN model consisting of two layers was designed using GCNConv layers. Node features were set up using the degree of each node.
4.3 Training
The model was trained using the Adam optimizer, and random labels were used to train the model.
Codage :
# -*- coding: utf-8 -*-
"""
Created on [date]
Author: [laktati]
"""
import pandas as pd
import torch
import torch.nn.functional as F
from torch_geometric.data import Data
from torch_geometric.nn import GCNConv
import networkx as nx
import matplotlib.pyplot as plt
import community # For Louvain method
file_path = 'dataset/facebook_combined.txt'
edges = pd.read_csv(file_path, delim_whitespace=True, header=None)
G = nx.Graph()
G.add_edges_from(edges.values)
edge_index = torch.tensor(edges.values.T, dtype=torch.long)
num_nodes = G.number_of_nodes()
class GNN(torch.nn.Module):
def __init__(self, in_channels, out_channels):
super(GNN, self).__init__()
self.conv1 = GCNConv(in_channels, 16)
self.conv2 = GCNConv(16, out_channels)
def forward(self, x, edge_index):
x = self.conv1(x, edge_index)
x = F.relu(x)
x = F.dropout(x, training=self.training)
x = self.conv2(x, edge_index)
return F.log_softmax(x, dim=1)
# إعداد ميزات العقد (features)
features = torch.tensor([[G.degree(i)] for i in range(num_nodes)], dtype=torch.float)
model = GNN(in_channels=1, out_channels=2)
optimizer = torch.optim.Adam(model.parameters(), lr=0.01)
def train():
model.train()
optimizer.zero_grad()
out = model(features, edge_index)
labels = torch.randint(0, 2, (num_nodes,), dtype=torch.long)
loss = F.nll_loss(out, labels)
loss.backward()
optimizer.step()
return loss.item()
num_epochs = 100
for epoch in range(num_epochs):
loss = train()
if epoch % 10 == 0:
print(f'Epoch {epoch}, Loss: {loss:.4f}')
model.eval()
with torch.no_grad():
out = model(features, edge_index)
preds = out.argmax(dim=1)
# رسم الشبكة
plt.figure(figsize=(12, 12))
pos = nx.spring_layout(G)
node_color = preds.numpy()
nx.draw(G, pos, node_color=node_color, with_labels=True, node_size=20, font_size=10, cmap=plt.get_cmap('coolwarm'))
plt.title("Social Network Graph with GNN Predictions")
plt.show()
partition = community.best_partition(G)
grouped_nodes = {}
for node, group in partition.items():
if group not in grouped_nodes:
grouped_nodes[group] = []
grouped_nodes[group].append(node)
print("Detected communities:")
for group, members in grouped_nodes.items():
print(f"Group {group}: {members}")
5. Results
Results were analyzed after training, where the model demonstrated its ability to classify users based on model predictions. The social network was also visualized with model predictions.
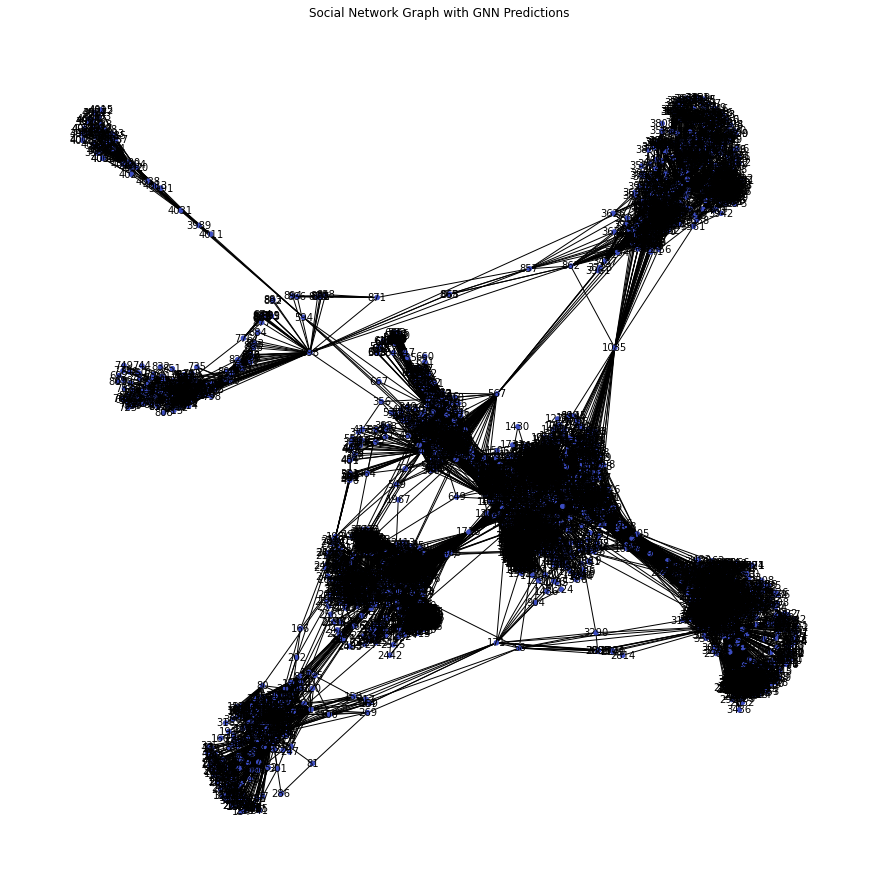
6. Discussion
The results indicate that GNNs can play a crucial role in social relationship analysis, aiding in community detection and enhancing understanding of the social structure of networks.
7. Conclusion
This research paper provides insight into the use of GNNs for social network analysis, opening avenues for future research to explore further applications in this field.
8. References
1. Kipf, T. N., & Welling, M. (2017). Semi-Supervised Classification with Graph Convolutional Networks. *ICLR*.
2. Newman, M. E. J. (2006). Modularity and community structure in networks. *Proceedings of the National Academy of Sciences*.
3. Zhang, S., & Zhan, X. (2019). A Survey of Graph Neural Networks: Models, Applications, and Future Directions. *arXiv*.